-
Homepage
-
lc3 program to read string and display it
Assignment
Problem Statement
For this assignment, you are to develop a LC-3 assembly program to read a string provided by theuser, store it in memory and redisplay it on the screen.
A skeleton code is provided to you. The code is missing some lines, which are indicated by the
following syntax:
;________ ;Add a line here to …
For instance, the first code you have to add asks:
;________ ;Add a line here to start your program at address x3000
You should replace this comment with the appropriate asm instruction, so that your program
originates at memory address x3000.
The expected program output is shown below.
;________ ;Add a line here to start your program at address x3000
;***************************************
;Part I: Initialize
;***************************************
;We allocated memory for the string already.
LEA r1, str1 ; addr of array to store string
;Why are we using LEA here and not LD or LDI?
AND r3, r3, #0 ; to store the size of the string/array
AND r2, r2, #0
;Prompt user to enter the string
LEA r0, prompt1
;PUTS writes a string of ASCII characters to the console display
;from the address specified in R0.
;Writing terminates with the occurrence of x0000
PUTS
;***************************************
;Part II: Read / Store the string
;***************************************
;
; Start reading characters
;
;GETC is same as TRAP x20: Reads a char and stores its ASCII code in R0
loop GETC ; Read a character.
;ASCII for newline/carriage return is LF and it is stored at #10
;________ ;Add a line here to check if the new char is a carriage return.
BRZ done
out ; echo character
STR r0, r1, #0
ADD r1, r1, #1 ; advance ptr to array
;________ ;Add a line here to increment size of the array
LD r6, EOL
ADD r4, r3, r6 ; check if we reached max length
BRN loop
;***************************************
;Part III: Display the string
;***************************************
done ;________ ;Add a line here to append NULL at the end of string
;Keep the label done. What is the ASCII char for NULL?
;Why are we adding NULL to the end of our string?
;________ ;Add a line here to add a carriage return to your string/array
out
LEA r0, str1
PUTS
HALT
prompt1 .STRINGZ “Enter a string of 30 characters or less. ”
str1 .BLKW 30 ; Allocate memory for chars to be stored
EOL .fill #-29 ; Limit of chars
.end
Solution
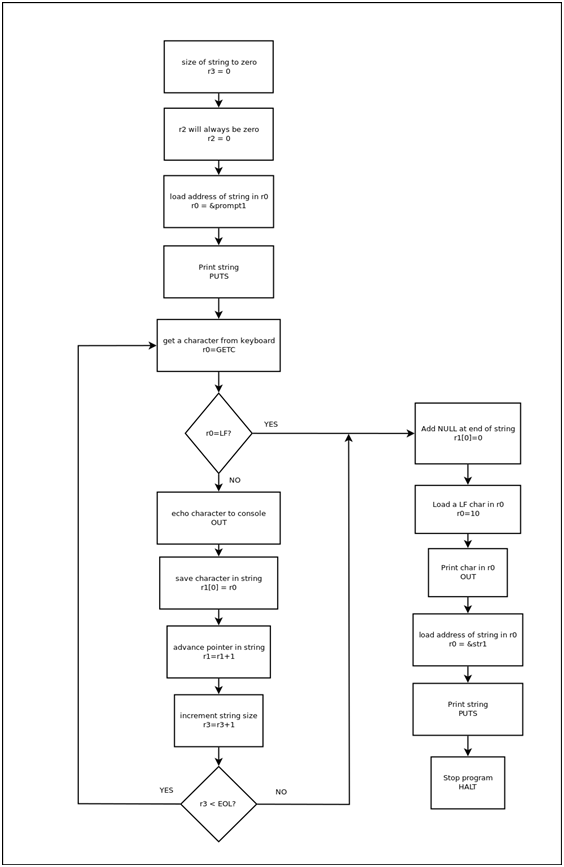
.ORIG x3000 ;Add a line here to start your program at address x3000
;***************************************
;Part I: Initialize
;***************************************
;We allocated memory for the string already.
LEA r1, str1 ; addr of array to store string
;Why are we using LEA here and not LD or LDI?
; LEA loads the starting address of the variable into r1, LD would load a value from the string and LDI would load a number
AND r3, r3, #0 ; to store the size of the string/array
AND r2, r2, #0
;Prompt user to enter the string
LEA r0, prompt1
;PUTS writes a string of ASCII characters to the console display
;from the address specified in R0.
;Writing terminates with the occurrence of x0000
PUTS
;***************************************
;Part II: Read / Store the string
;***************************************
;
; Start reading characters
;
;GETC is same as TRAP x20: Reads a char and stores its ASCII code in R0
loop GETC ; Read a character.
;ASCII for newline/carriage return is LF and it is stored at #10
ADD r4,r0,#-10 ;Add a line here to check if the new char is a carriage return.
BRZ done
out ; echo character
STR r0, r1, #0
ADD r1, r1, #1 ; advance ptr to array
ADD r3, r3, #1 ;Add a line here to increment size of the array
LD r6, EOL
ADD r4, r3, r6 ; check if we reached max length
BRN loop
;***************************************
;Part III: Display the string
;***************************************
done STR r2, r1, #0 ;Add a line here to append NULL at the end of string
;Keep the label done. What is the ASCII char for NULL?: 0
;Why are we adding NULL to the end of our string? PUTS prints all characters in a string until a NULL is found
ADD r0,r2,#10 ;Add a line here to add a carriage return to your string/array
out
LEA r0, str1
PUTS
HALT
prompt1 .STRINGZ “Enter a string of 30 characters or less. ”
str1 .BLKW 30 ; Allocate memory for chars to be stored
EOL .fill #-29 ; Limit of chars
.end
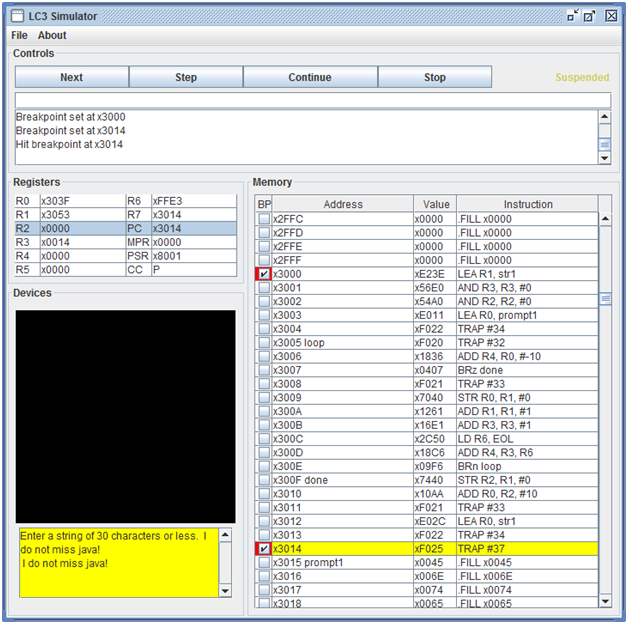