Simple Text Encryption in Java: A Beginner's Guide
May 26, 2023
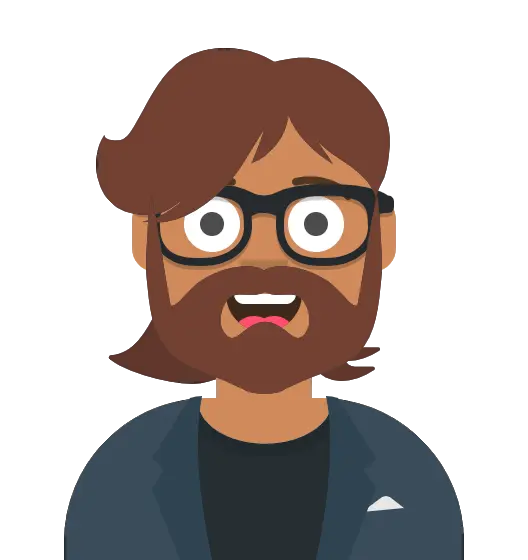
Diego White
United States
Java
He is a highly skilled Java developer with over 10 years of experience. He specializes in encryption techniques and has a deep understanding of implementing secure systems.
Do you Need assistance in assignments on simple text encryption in Java? Our team of experienced developers is here to help with your Programming Assignments. We have a strong background in encryption techniques and can guide you through the implementation process. Get expert support to ensure the security of your sensitive data. Contact us today!
- In today's interconnected world, where information is exchanged at an unprecedented rate, ensuring the security and confidentiality of sensitive data has become a top priority. Encryption, the process of converting plain text into a coded form known as cipher text, is critical in preventing unauthorized access to information. In this blog, we will delve into the fascinating world of encryption, with a particular emphasis on simple text encryption in the context of Java programming.
- Encryption is a strong defense mechanism that prevents data from being intercepted, tampered with, or understood by unauthorized individuals. Organizations and individuals can protect their private communications, sensitive personal data, financial transactions, and other critical information from prying eyes by using encryption techniques. Java emerges as a formidable tool for implementing encryption systems due to its robust and versatile features.
- One of the key benefits of using Java for encryption is its platform independence. Java programs can run on any platform that has a Java Virtual Machine (JVM) installed, removing the need for recompilation or modification when deploying code across operating systems. Because of its versatility, Java is an excellent choice for developing encryption systems that must be deployed across multiple platforms.
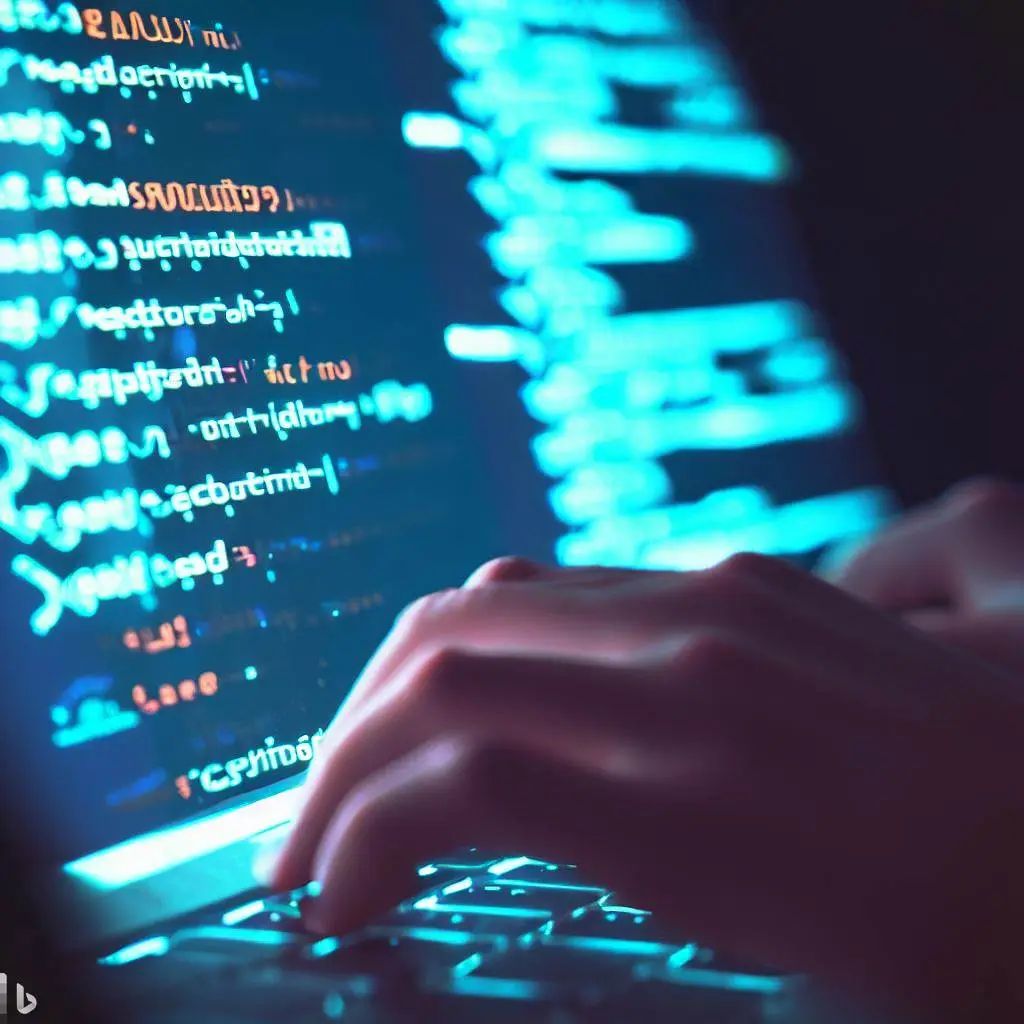
- Java includes a comprehensive set of security features and libraries that make it easier to implement encryption algorithms. The Java Cryptography Architecture (JCA) and Java Cryptography Extension (JCE) provide a comprehensive set of cryptographic algorithms, including symmetric and asymmetric encryption, digital signatures, hash functions, and key management. These standardized libraries ensure compatibility and interoperability with other systems and programming languages, allowing Java encryption solutions to be easily integrated into existing software infrastructure.
- Furthermore, Java has a thriving and active community of developers and security experts. The availability of encryption-related resources, forums, and documentation in Java makes it easier for developers to gain knowledge, seek assistance, and stay up to date on the latest encryption techniques and best practices. The active community support encourages collaboration and the sharing of insights, allowing developers to improve their encryption implementations and effectively address potential vulnerabilities.
- Java's ability to optimize performance also contributes to its suitability for encryption tasks. Java's Just-In-Time (JIT) compiler allows it to dynamically optimize code execution, improving overall performance. Encryption operations can be computationally intensive, but Java's ability to optimize code and leverage hardware acceleration, such as native libraries, enables encryption algorithms to be executed efficiently.
- Another advantage of using Java for encryption is its scalability and extensibility. Because of Java's modular architecture and object-oriented nature, developers can create flexible and scalable encryption systems. This is especially useful when integrating encryption with other software components or dealing with large amounts of data. Because of Java's flexibility, encryption solutions can adapt to changing security requirements and integrate seamlessly into complex software ecosystems.
- Aside from these technical advantages, Java's compliance with various security standards strengthens its position as a preferred choice for encryption implementations. Specific security standards and regulations often require the use of encryption in industries and organizations. Java's encryption capabilities are compliant with industry standards like FIPS and PCI DSS, ensuring that encryption solutions built in Java meet stringent compliance requirements.
Encryption Fundamentals:
The process of converting plain text into cipher text in order to prevent unauthorized access to the original message is known as encryption. Encryption's primary goal is to make the cipher text unreadable to anyone who does not have the decryption key. This ensures the information's confidentiality and integrity. To transform data, encryption algorithms use mathematical operations and cryptographic techniques.
Algorithms for Encryption:
There are numerous encryption algorithms available, each with its own set of characteristics and security levels. In this blog, we will look at the Caesar cipher, a simple but effective encryption algorithm. The Caesar cipher is a substitution cipher that works by shifting each character in plain text by a predetermined number of positions. While the Caesar cipher is simple to understand and use, it is a weak encryption method that is not suitable for securing highly sensitive data.
Let's start with some of the benefits of using Java for encryption:
Independence of Platform:
Java is a platform-independent programming language, which means that Java code can run on any platform that has a Java Virtual Machine (JVM) installed. This makes Java a versatile choice for encryption because the encryption code can be written once and deployed on multiple platforms without requiring major changes.
Strong security features include:
Java has a comprehensive set of security features and APIs (Application Programming Interfaces) that make encryption and cryptography easier. The Java Cryptography Architecture (JCA) and Java Cryptography Extension (JCE) provide a diverse set of cryptographic algorithms and protocols, ensuring that robust encryption options are available.
Libraries that have been standardised:
Java includes encryption libraries, such as the javax.crypto package, which includes classes and methods for implementing encryption algorithms. These libraries adhere to industry standards and specifications, ensuring system and language compatibility and interoperability.
Support from the Community:
Because Java has a large and active developer community, there are numerous resources, forums, and documentation available to assist with encryption-related issues. This community support makes it easier to find solutions, share knowledge, and keep up to date on the most recent encryption techniques and best practices.
Performance Enhancement:
The Just-In-Time (JIT) compiler in Java optimizes code execution, resulting in high performance. While encryption operations can be computationally intensive, Java's ability to optimize code and leverage hardware acceleration (via native libraries, for example) can improve overall encryption performance.
Extensibility and scalability:
Java's object-oriented nature and modular architecture make it highly scalable and extensible. This is useful when developing encryption systems that may need to integrate with other components or handle large amounts of data. Because of Java's flexibility, complex encryption solutions can be created that can evolve and adapt to changing security requirements.
Error Management and Exception Management:
Exceptions in Java provide robust error handling mechanisms. This enables developers to gracefully handle errors and exceptions that may occur during encryption operations, ensuring the encryption process's reliability and integrity.
Security Standards Compliant:
Encryption is required by many industries and organizations' security standards and regulations. Java's encryption capabilities are compliant with a variety of security standards, including FIPS (Federal Information Processing Standards) and the Payment Card Industry Data Security Standard (PCI DSS), making it a good choice for industries that require strict compliance.
Overall, Java provides a solid foundation for implementing encryption systems, combining platform independence, comprehensive security features, community support, performance optimization, scalability, and security standard compliance. Because of these benefits, Java is a popular and dependable choice for encryption applications.
Caesar's Cipher Algorithm:
The Caesar cipher algorithm works in the following manner:
Enter the following plain text:
Begin by taking the user's input for the text to be encrypted. This can be any character sequence, including letters, numbers, and symbols.
Set the shift value:
Determine how many positions each character should be moved. This value can be either positive or negative. A positive shift value causes the characters to move to the right, while a negative shift value causes them to move to the left.
Encrypt the plain text as follows:
Iterate through the plain text, applying the shift operation to each character. If the character is a letter, it will be shifted within the alphabet's range, wrapping around as needed. Keep the characters' cases during the encryption process. Non-alphabetic characters do not change.
Produce the cipher text:
As a final result, show the user the encrypted text. The cipher text will be a transformed version of the original plain text, with each character shifted by the shift value specified.
Using the Caesar Cipher in Java:
We can use the steps outlined above to implement the Caesar cipher in Java. The encryption process is demonstrated in the provided code snippet. It should be noted, however, that this implementation is for educational purposes only and should not be used for secure encryption in production environments.
public class CaesarCipher {
public static String encrypt(String plainText, int shift) {
StringBuilder cipherText = new StringBuilder();
for (int i = 0; i < plainText.length(); i++) {
char currentChar = plainText.charAt(i);
if (Character.isLetter(currentChar)) {
char base = Character.isUpperCase(currentChar) ? 'A' : 'a';
char encryptedChar = (char) ((currentChar - base + shift) % 26 + base);
cipherText.append(encryptedChar);
} else {
cipherText.append(currentChar);
}
}
return cipherText.toString();
}
public static void main(String[] args) {
String plainText = "Hello, World!";
int shift = 3;
String cipherText = encrypt(plainText, shift);
System.out.println("Plain Text: " + plainText);
System.out.println("Cipher Text: " + cipherText);
}
}
The encrypt method in the provided code takes the plain text and the shift value as parameters. It iterates through the plain text, checking each character to see if it is a letter and then performing the necessary shift operation based on the shift value. The cipher text that results is stored in a StringBuilder and returned as a string.
Conclusion:
In this blog, we looked at the idea of simple text encryption in Java. Encryption is a critical technique for protecting sensitive data from unauthorized access. We talked about the Caesar cipher algorithm, which is a simple but effective encryption method. The Caesar cipher works by shifting each character a predetermined number of positions. It should be noted, however, that the Caesar cipher is considered a weak encryption method and should not be used to secure highly sensitive data.
More advanced encryption algorithms, such as AES (Advanced Encryption Standard) and RSA (Rivest-Shamir-Adleman), offer greater security and are widely used in modern applications. Furthermore, encryption is a broad field with many advanced techniques and best practices to consider. This blog is a good place to start learning about simple text encryption in Java. Remember to always broaden your knowledge, stay up to date on the latest encryption standards, and use strong encryption methods to ensure the confidentiality and integrity of your data in the digital world.