Advanced Techniques for Scala Assignment Problem Solving
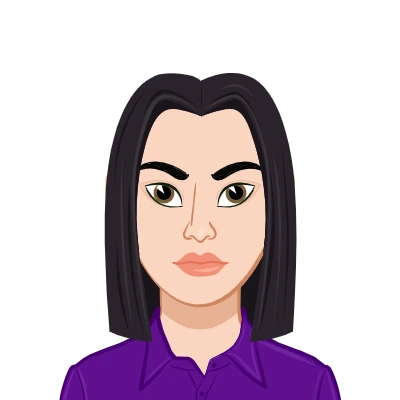
Scala is a powerful programming language that combines the object-oriented and functional programming paradigms. It has become very popular in recent years because it is easy to use, short, and can be expanded. When it comes to solving Scala assignment problems, knowing a lot about advanced techniques can greatly improve your problem-solving skills and help you write code that is both efficient and beautiful. In this blog post, we'll look at a few advanced techniques that can help you do good work on difficult Scala assignments.
Pattern Matching and Case Classes
Pattern matching in Scala is a flexible feature that lets you match complex data structures to certain patterns. It helps a lot when working with algebraic data types or data structures that loop back on themselves. On the other hand, case classes make it easy to define data structures that can't be changed. By using pattern matching and case classes together, you can make code that is short and easy to read and use to solve different assignment problems.
Exhaustive Pattern Matching
Exhaustive pattern matching makes sure that you've thought of everything and that there's no room for unexpected behavior. It helps you find possible errors at the time of compilation and makes your code more stable and easy to work with. With Scala's powerful syntax for pattern matching, it's easy to break down data structures and pull out values based on certain patterns.
For example, when working with lists, you can use pattern matching to deal with empty lists, lists with only one item, and lists with more than one item. This lets you handle different situations in the right way and keep runtime errors from happening.
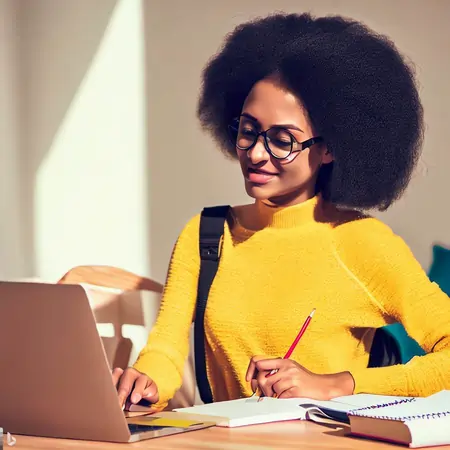
Pattern Matching with Guards
When you use guards with pattern matching, you can add more conditions to the patterns. This gives you the freedom to deal with different situations based on certain conditions. This makes your code more expressive and flexible. Guards can have Boolean expressions that make the pattern-matching behavior even more precise.
For example, if you are working with a case class that represents a person's age, you can use pattern matching with guards to handle certain age ranges differently. This lets you use different logic depending on whether or not the age is in a certain range or meets a certain condition.
Higher-Order Functions and Functional Programming
Scala is based on functional programming, and using higher-order functions can make your code much easier to understand and more modular. If you treat functions like they are first-class citizens, you can pass them as arguments, get them back as results, and put them together to solve hard problems in a more elegant way.
Function Composition
Function composition enables you to combine multiple functions into a single function. By breaking up complex operations into smaller, composable parts, this technique encourages code reuse, cuts down on duplication, and makes code easier to read.
To put together functions in Scala, you can use the 'compose' and 'andThen' methods. With the "compose" method, you can chain two functions together to make a new function. The output of the second function becomes the input of the first function. The 'andThen' method is the same, but it works in the opposite order.
Using function composition, you can make pipelines of operations, each of which is a separate function, and easily use them to solve your programming assignment problems. This not only makes the code easier to understand, but it also makes it easier to maintain and test.
Currying
Currying is the process of turning a function that takes many arguments into a series of functions that each take only one argument. With this method, you can only use some of the arguments, making functions that can be used in different situations.
Currying lets you make functions in Scala that only take some of the arguments and return a new function that takes the rest. This enables you to create more flexible and reusable code. For example, say you have a function that takes two numbers and does a math operation on them. By currying the function, you can make addition, subtraction, multiplication, etc. functions that are already set up with one of the arguments. This means that you can use these specialized functions in different parts of your code without having to write the same logic more than once.
Higher-Order Functions in Collections
Scala collections have a lot of higher-order functions like'map', 'filter','reduce', and 'fold'. Using these functions, you can express complex data transformations in a way that is clear and quick.
The'map' function applies a change to each item in a collection. This makes a new collection of values that have been changed. This is helpful when you want to perform a certain operation or change on each item in a collection.
With the 'filter' function, you can choose which items from a collection to keep based on a given predicate. It is useful for getting rid of things that don't meet certain requirements.
With the'reduce' and 'fold' functions, you can combine parts of a collection into a single result. They can be used to figure out totals or summaries of data.
By combining these higher-order functions with lambdas or anonymous functions, you can describe complex data operations in a clear and concise way. This makes your code easier to read and maintain.
Concurrency and Parallelism
Scala has strong abstractions for concurrent and parallel programming, which makes it a great language for solving problems that need to use multi-core processors efficiently. Your Scala assignments will run much faster if you understand these ideas and use the right techniques for concurrency and parallelism.
Futures and Promises
Futures and promises are Scala structures that make it easier to program in an asynchronous way. By using these abstractions, you can do operations that don't block and handle the results asynchronously. This lets you use system resources more efficiently.
A future is a way to talk about a calculation that may or may not be done yet. You can create a future to do a task that takes a long time, and the result of the task will be available at a later time. This lets you keep working on other things at the same time without having to wait for the result.
A promise is a single-assignment container that can be written to and holds a value that is not yet ready. You can make a promise, do a calculation, and then use the result to keep the promise once it is ready. The promise that has been kept can then be used to get the result. By combining futures and promises, you can describe computations that are happening at the same time, handle dependencies between tasks, and make sure they are all done at the same time.
Parallel Collections
Parallel collections let you process data at the same time by splitting the work between multiple threads or processors. They make it easy to take advantage of the full power of modern hardware and make your Scala code run faster.
Parallel collections in Scala add to the features of regular collections by letting you run operations on their elements at the same time. Existing code can be made to work in parallel with only minor changes, such as switching from regular collections to parallel collections.
Parallel collections handle parallelism and the distribution of tasks across threads or processors on their own, optimizing the execution based on the resources available. This lets you use multi-core processors to your advantage and speed up how your Scala assignments run.
Error Handling and Exception Handling
Handling errors correctly is a must if you want your Scala code to be strong and reliable. Understanding the different ways to handle errors and the best ways to do so can help you plan for and deal with errors well, making sure that your assignments are resilient and can handle mistakes.
Option and Either
Scala's 'Option' and 'Either' types are powerful ways to deal with values that could be wrong. By using them correctly, you can get rid of null pointer exceptions and deal with errors in a way that is both functional and clear.
The 'Option' type stands for a value that may or may not exist. It can be used to figure out what to do when a value is missing. Instead of returning null, you can wrap the value in 'Option' to show if it is present ('Some') or not present ('None'). This makes you handle both cases explicitly, which makes your code safer and easier to read.
The 'Either' type stands for a value that can be either a success or a failure. It is often used to deal with operations that can go wrong. Convention says that the right side of the "Either" means success and the left side means failure. This lets you store more information about errors along with the result.
By using the 'Option' and 'Either' types, you can make sure your Scala assignments have better error handling, avoid unexpected null values, and give clear and explicit error messages.
Conclusion
You can become a better Scala developer if you use advanced techniques like pattern matching, higher-order functions, concurrency and parallelism, and the right way to handle errors. With these techniques, you can solve complex homework problems better, write cleaner code, and make applications that are strong and scalable. Adopt these methods, practice often, and keep looking into the many things Scala can do to improve your problem-solving skills and become an expert at solving Scala assignment problems.