Fibonacci function/Subroutine Using C Programming Language
In this sample C programming assignment a subroutine asuAdd is given. Students are required to write a function in C Programming language using the subroutine asuAdd to calculate huge Fibonacci numbers.
The prototype/signature for the function is given as follows : –
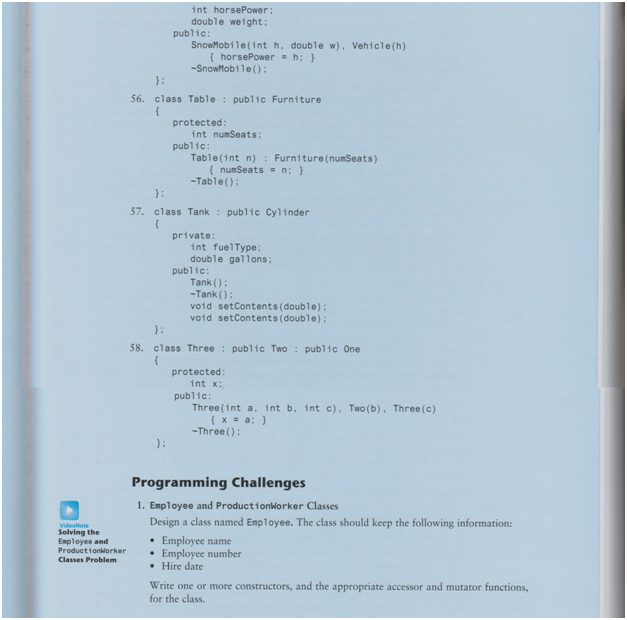
n is the Fibonacci number that needs to be calculated. The series of Fibonacci number starts from values 0, 1; and n is to be calculated.
SOLUTION : –
#include
#define MAX_SIZE_TEST 1000
typedef unsigned int bigNumTest[1 + MAX_SIZE_TEST];
typedef unsigned int bigNumN[];
int asuAdd(bigNumN bigN0P, const bigNumN bigN1P, unsigned int maxN0Size);
void halt()
{
}
/*
* function: asuFibonacci
*
* n is the Fibonacci number that you would like to calculate. (Our Fibonacci
* numbers start with f0 = 0, f1 = 1, etc., and we would like to calculate fn.)
*
* The return value of the function is either n, or the largest Fibonacci number
* that could be accurately calculated before overflow occurred.
*
* maxSize is an integer that specifies how many valid words can be stored in the
* bigNumN numbers.
*
* bNP is a pointer to a pointer to a bigNumN number holding the Fibonacci number
* indicated by the return value. bNP will either point to static array bNa or
* static array bNb.
*
*/
int asuFibonacci(int n, int maxSize, bigNumN **bNP) {
// **** modify below and fill in code and ensure proper operation including return value. ***
static bigNumTest bNa = {1,0,0}; // initial fibonacci (0) = 0
static bigNumTest bNb = {1,1,0}; // initial fibonacci (1) = 1
int i;
if (n == 0)
{
*bNP = &bNa;
return 0;
}
else if (n == 1)
{
*bNP = &bNb;
return 1;
}
*bNP = &bNb;
for (i = 2; i <= n; i++)
{
if (!(i % 2)) // If it’s even
{
if (asuAdd( bNa, bNb, maxSize))
{
// if overflow, return last i
n = i – 1;
break;
}
*bNP = &bNa;
}
else // if it’s odd
{
if (asuAdd( bNb, bNa, maxSize))
{
// if overflow, return last i
n = i – 1;
break;
}
*bNP = &bNb;
}
}
return n;
}
int main() {
bigNumN *fibResP = NULL;
int i = asuFibonacci(1000000, MAX_SIZE_TEST, &fibResP);
halt();
return i;
}
// or move this to the assembly file
void _exit(int status) {
volatile int x; // work around problem with xilinx system debugger.
loop:
x++;
goto loop;
}