Common Mistakes to Avoid in Scala Programming Assignments
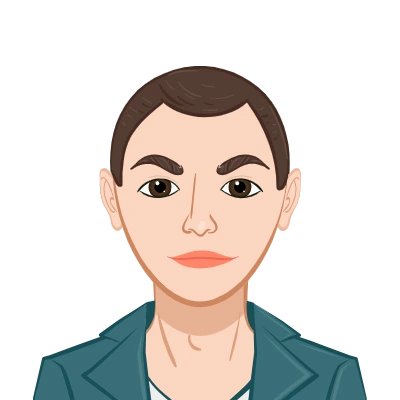
Even the most experienced developers can make common mistakes when working on Scala programming assignments. These mistakes can slow them down and lower the quality of their work. In this blog post, we'll talk about some of these mistakes and give you helpful advice on how to avoid them. By knowing about these mistakes, you can improve your Scala programming skills and make sure your assignments turn out well. Let's dive in!
Mistakes Related to Syntax and Semantics
There are common mistakes in syntax and meaning that Scala developers should be aware of. If you don't understand Scala's type system, it can lead to bugs and strange behavior. Using implicit conversions in the wrong way can cause problems you didn't expect. Also, runtime errors can happen if you don't understand how important pattern matching is or if you make pattern-matching expressions too complicated. By keeping these syntax and semantics mistakes in mind, developers can improve the quality of their code and avoid making these common mistakes in their Scala programming assignment help.
Neglecting to Understand Scala's Type System
Scala has a powerful type of system that lets you write programs that are both flexible and expressive. But mistakes can happen if you don't understand its subtleties. Some of the most common mistakes are:
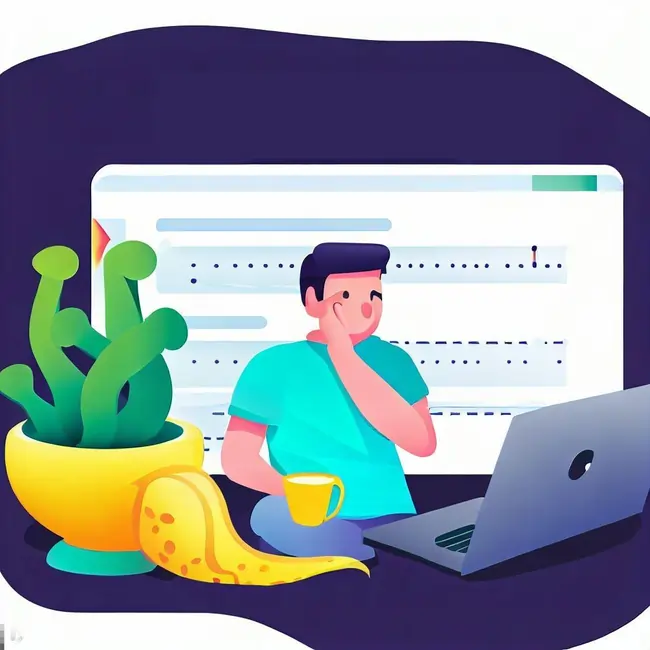
- Inconsistent or wrong use of type annotations: If you don't give correct type annotations, type inference errors or strange behaviors can happen. Always make sure to explicitly label types when you need to.
- Misunderstanding implicit conversions: Scala's implicit conversions can be a powerful tool, but if they are used wrong, they can also cause unexpected behavior. Use implicit conversions with care, and make sure they are used consistently and in a way that is clear and easy to understand.
Ignoring the Importance of Pattern Matching
Pattern matching is an important part of Scala that makes it possible to write code that is both clear and concise. But many developers don't understand how important it is and miss out on its benefits. Some common mistakes in pattern matching are:
- Runtime errors can happen if you don't cover all possible cases or give the wrong patterns. Make sure that your pattern-matching expressions deal with every possible case.
- Making pattern-matching expressions too hard to understand. Pattern matching can handle complicated situations, but expressions should be kept simple and easy to understand. Don't put patterns inside of each other or use patterns that are too hard to understand.
Mistakes in Functional Programming
Functional programming in Scala is a great way to write code that is clear and easy to understand. But making certain mistakes can make functional programming principles less useful. One common mistake is not fully accepting that things can't change. If you don't take care of immutable data, it can lead to unexpected bugs and make it harder to understand code. Overusing complex higher-order functions like nested maps and filters is another mistake that can lead to code that is hard to read and keep up to date. NullPointerExceptions can also be caused by bad handling of null values or bad use of Option types. To avoid these mistakes, functional Scala programming should aim for simplicity, keep immutability, and deal with optionality well.
Mutating Variables in an Immutable Context
Scala's main idea is that things shouldn't change, but it's easy to use mutable variables in situations where they shouldn't. This can cause bugs and strange things to happen. Think about the following errors:
- Reassigning variables declared as 'val': You can declare variables as 'val' in Scala to make sure they don't change. But changing these variables goes against the idea that things shouldn't change. Make sure that 'val' variables are never reassigned.
- Changing collections directly: It is important to avoid changing collections directly when working with them, especially in functional programming. Instead, use transformation operations like'map', 'filter', and 'fold' to make new collections.
- Using mutable state too much: In functional programming, you should try to use mutable state as little as possible. If you depend too much on state that can change, it can make your code hard to understand and test. Try to write pure functions with as few side effects as possible.
Insufficient Error Handling in Functional Code
With techniques like "Option," "Either," and "Try," functional programming in Scala makes it easier to deal with errors in the right way. If you don't handle errors properly, your program could crash without warning or act in the wrong way. Some common mistakes are:
- Ignoring exceptions: Don't catch exceptions without taking care of them properly. By swallowing exceptions, you can hide important information and make it harder to find bugs. Handle exceptions explicitly with 'Try' or 'Either' to make error messages that make sense.
- Ignoring the 'Option' type: The 'Option' type in Scala is made to handle nullable values and force explicit handling of possible nulls. Null pointer exceptions can happen if you don't pay attention to the 'Option' type. Use methods like 'getOrElse' or pattern matching to handle 'Option' values in the right way.
- Not having a backup plan: When using "Option," "Either," or "Try," make sure you have a backup plan or an error message ready in case something goes wrong. If these situations aren't handled, the program might act in a way that wasn't expected.
Mistakes in Object-Oriented Programming
Developers often make mistakes in object-oriented programming that make it harder to maintain and add to their code. Too much inheritance is a common mistake. Even though inheritance can be useful, using it too much can lead to classes that are too tightly linked and code that is hard to understand and change. Composition, where objects are made up of smaller, reusable parts, is better than inheritance. Another mistake is method overriding which doesn't make sense. When overriding methods in subclasses, it's important to follow the contracts set up in the superclass to make sure things work right and keep the Liskov Substitution Principle in place.
Misusing Mutable State
Scala can be used for both object-oriented programming and functional programming. To avoid common mistakes when using object-oriented programming, it's important to handle mutable states the right way. Think about these wrong uses:
- Sharing state that can change without synchronization: When multiple threads access a shared state that can change at the same time, they should use synchronization tools like locks or atomic operations. Failure to do so can lead to race conditions and data inconsistencies.
- Inconsistent use of 'var' and 'val': Scala provides both mutable ('var') and immutable ('val') variables. If you don't use them right, you might get confused or have unintended side effects. Use 'val' whenever you can to prevent changes.
Overcomplicating Class Hierarchies
In object-oriented programming, if class hierarchies aren't set up carefully, they can get complicated and hard to manage. Avoid these mistakes:
- Using inheritance too much: inheritance can be a powerful tool, but using it too much can lead to code that is hard to understand and keep up to date. Whenever possible, it's better to make your own things than to inherit.
- Overriding methods in different ways: When overriding methods in subclasses, make sure that the new methods still follow the rules set by the superclass. If you don't do this, unexpected things can happen, which goes against the Liskov Substitution Principle.
Mistakes in Scala Collections
To write code that works well and quickly, you need to know how Scala collections work. Using the wrong collection type for certain operations is a common mistake that can slow things down. Memory overhead can also be caused by inefficient use of collection operations, such as making unnecessary intermediate collections. Another mistake is not thinking about how long operations take, which could affect performance with large datasets. Developers can avoid these pitfalls and use Scala collections to their full potential by being aware of the types of collections, optimizing operations, and understanding how time complexity works.
Not Understanding the Performance Implications of Collection Operations
Scala has a large number of collection classes that can be used in different ways. But if you don't know how these operations work, you might not be able to do them as well as you could. Think about these errors:
- Using the wrong collection type: Different collection types work better for certain operations than others. For example, if you use a "List" for frequent random access, the performance can be bad. Learn the pros and cons of each type of collection and choose the one that works best for your needs.
- Using collection operations in an inefficient way: Some collection operations, like "filter" and "map," make new collections. When you do multiple operations on a large collection one after the other, it can create unnecessary intermediate collections and take up extra memory. Use lazy collections or methods like 'view' or 'iterator' to avoid using too much memory.
- Not being aware of time complexity: Each collection operation takes a different amount of time to do. If you don't think about the time complexity, your performance might not be as good as it could be. When working with large datasets, be aware of how long operations like "contains," "find," and "groupBy" take.
Mistakes in Error Handling and Exception Management
One common mistake in handling errors and managing exceptions is to catch too many of them. When exceptions are caught at a higher level without being specific, it can hide problems that are really happening and make it hard to debug. It's important to catch exceptions at the right level and handle errors in a way that fits each situation. Another mistake is not using functional alternatives to handling exceptions in Scala, like Either and Try. By using these building blocks, developers can improve error handling and make their code more reliable. Also, it's important to set up the right logging and error reporting systems to make troubleshooting and problem solving easier.
Relying Too Heavily on Exception Handling
Scala does support exceptions, but using them too much can make code harder to understand and maintain. Think about these mistakes when it comes to handling errors and managing exceptions:
- Catching too many exceptions: Catching exceptions at a higher level without being specific enough can hide bugs and make them hard to find and fix. It's important to handle errors in a targeted way and catch exceptions at the right level.
- Not using the Either and Try types in Scala: Scala has functional alternatives to handling exceptions, such as "Either" and "Try," which let you handle errors more clearly. If you don't use these constructs, your error management might not be as good.
- Not logging and reporting errors well: When exceptions happen, it's important to have a strong logging system in place. If you don't log exceptions or don't give enough information, it can slow down troubleshooting and make it harder to find and fix problems.
Mistakes in Concurrency and Parallelism
If you don't use concurrency constructs the right way, you could end up with subtle bugs and performance problems. When multiple threads share mutable state without proper synchronization, data races and inconsistent behavior can happen. It is very important to use synchronization mechanisms or immutable data structures to protect shared mutable state. Also, using parallelism without thinking can lead to extra work and inefficient use of resources. Find the parts of your code that can be run in parallel and use the right tools, like parallel collections or Future.sequence, to make parallelism work well and improve performance.
Incorrect Use of Concurrency Constructs
Scala has powerful concurrency constructs like 'Future' and 'Actor' that make it easier to write programs that run in parallel or at the same time. But wrong usage can cause small bugs and performance problems. Think about these mistakes:
- Sharing mutable state between multiple threads: If multiple threads try to access shared mutable state at the same time without proper synchronization, this can lead to data races and strange behavior. Make sure that synchronization mechanisms or immutable data structures are used to protect shared mutable state.
- Parallelism that doesn't work well: Parallelism can improve performance by a lot, but if it's used without thinking, it can cause extra work and waste resources. Find the parts of your code that can be run in parallel and use the right tools, such as parallel collections or "Future.sequence," to make parallelism work well.
Lack of Error Handling in Concurrent Code
When working with code that runs in parallel, it's important to handle errors in the right way to make sure the program works right. Think about these mistakes when it comes to handling errors in parallel situations:
- Not handling exceptions in asynchronous code: It's very important to handle exceptions correctly when working with asynchronous constructs like "Future." Unhandled exceptions can cause futures to fail silently, which can cause the program to act in a way that was not expected.
- Not taking care of "race conditions" well: Code that runs in parallel can cause "race conditions," in which the result depends on the order in which operations are run. Failing to deal with race conditions can lead to wrong results or behavior that is hard to predict. For correctness, use the right synchronization tools, like locks or atomic operations.
Conclusion:
By being aware of these common mistakes and using the best practices in this blog post, you can improve your Scala programming skills and turn in high-quality assignments. Remember to pay attention to syntax and semantics, use functional programming principles, and use object-oriented programming well. If you avoid these mistakes, you will not only get better at Scala, but you will also become a better and more reliable developer. Good luck coding!