How to Write an Assignment on Multithreaded Huffman Decoder in C++
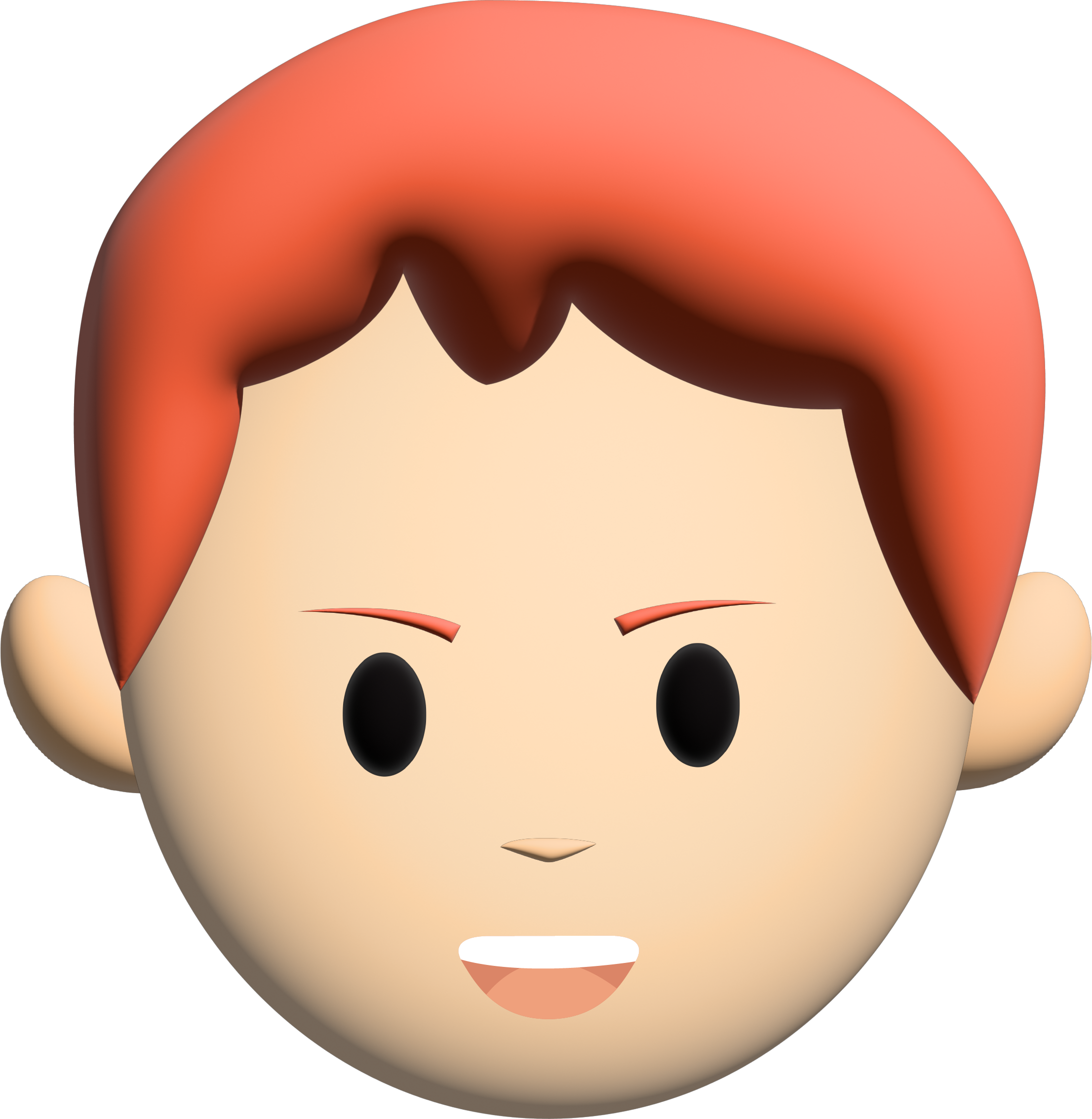
Multithreading is a powerful technique used in computer science that enables programmes to carry out multiple tasks at once. Applications that need to manage a lot of data or carry out intricate calculations can perform a lot better thanks to it. With the help of a popular data compression algorithm called Huffman coding, data can be compressed by using fewer bits to represent it. It takes an in-depth knowledge of both multithreading and the Huffman coding algorithm to write an assignment on a multithreaded Huffman decoder in C++.
In this blog post, we'll go over the crucial procedures for creating and putting a multithreaded Huffman decoder in C++. The Huffman coding algorithm and the fundamentals of multithreading will be covered first. The process of creating a multithreaded decoder will then be described in detail, including reading the input, creating threads, creating the Huffman tree, decoding the data, splitting the input data, decoding the data in parallel, and combining the results. We'll finish up by talking about testing and debugging strategies that can help you make sure your multithreaded decoder operates properly and effectively.
You can produce an impressive assignment that showcases your programming abilities and understanding of multithreading and data compression by following the instructions provided in this blog. Learning how to create a multithreaded Huffman decoder in C++ can help you develop your coding abilities and take on more challenging projects, whether you are a professional programmer or a student. Let's begin by learning how to write a C++ assignment on a multithreaded Huffman decoder.
Understanding Multithreaded Huffman Decoder
You must first comprehend the fundamentals of both multithreading and the Huffman coding algorithm in order to write an assignment on a multithreaded Huffman decoder in C++. Through the use of the multithreading technique, a programme can run multiple threads concurrently, enhancing performance and responsiveness. A lossless data compression algorithm called Huffman coding employs variable-length codes to represent data in a more condensed manner.
Reading the input data and creating a Huffman tree are the first steps in creating a multithreaded Huffman decoder. The input data is decoded using the Huffman tree, which also displays the character frequencies in the data. You can begin decoding the data by traversing the Huffman tree after it has been constructed and swapping out the codes for the corresponding characters.
You can create multiple threads and distribute the input data among them to speed up the decoding process. The data can then be independently and concurrently decoded by each thread, one at a time. You must synchronise the threads' access to shared resources, like the Huffman tree and the decoded output, to make sure that everything runs smoothly.
You can design and implement a multithreaded Huffman decoder in C++ by being familiar with these fundamental ideas. The steps of the process will be covered in greater detail, and the best methods and procedures for creating a high-caliber decoder will be examined in the sections that follow.
How Huffman Coding Works
- The data compression method known as Huffman coding gives each symbol in a message a variable-length code. Based on how frequently a symbol appears in the message, a code is created. The code gets shorter the more frequently used a symbol is.
- The encoder creates a Huffman tree from the input symbols' frequency table in order to compress the data. In the Huffman tree, which is a binary tree, each leaf node stands in for a symbol, and the path leading from the root to a leaf node denotes the code that has been assigned to that symbol.
- The encoder moves through the Huffman tree, appending the bits of the code to the output stream, from the root to the leaf node corresponding to the input symbol. The decoder traverses the Huffman tree from the root to the leaf node corresponding to the code in order to decode the data by reading the bits from the encoded data.
Multithreading
A programme can divide its execution into multiple threads that can run simultaneously using the multithreading technique. The operating system arranges for the threads to run on the available CPU cores, and each thread is capable of carrying out a different task.
By dividing the workload into numerous parallel-running threads in a multithreaded programme, you can improve performance. Due to the need to synchronise the threads in order to prevent race conditions and deadlocks, multithreading can be difficult.
Multithreading in C++
Using the C++11 standard's thread library, you can implement multithreading in C++. In order to create a thread, you must first define the function that will run on it and then create an object called a thread that will carry out the function.
You can make use of mutexes, condition variables, and atomic variables to synchronise the threads and prevent race conditions. A shared resource is locked using mutexes so that only one thread can access it at once. In order to signal and wait for a specific condition to be satisfied, condition variables are used. Atomic operations on shared variables are carried out using atomic variables.
Designing a Multithreaded Huffman Decoder
The overall architecture and data structures must be carefully taken into account when designing a multithreaded Huffman decoder. How the input data is distributed among the threads is one of the important design choices. You might need to start more threads to process the data, depending on its size and complexity.
The synchronisation of the threads is a further crucial factor. The threads must be in sync in order to access shared resources like the Huffman tree or the output buffer without interfering with one another. Locks, semaphores, or mutexes are just a few examples of synchronisation methods that can be used to accomplish this.
The decoder's functionality and efficiency can both be significantly impacted by the choice of data structures. For instance, the decoding procedure can be significantly sped up by storing the Huffman tree's nodes in a priority queue or a heap. Similar to this, storing the output data in a queue or a circular buffer can cut down on data copying costs and boost performance.
In general, designing a multithreaded Huffman decoder necessitates a thorough comprehension of the issue and the resources at hand. You can build a decoder that is effective, dependable, and scalable by carefully taking into account the architecture, data structures, and synchronisation strategies.
Step 1 - Read the Input
Reading the input file containing the encoded data is the first step. The C++ standard input/output streams can be used for this. It's crucial to deal with any errors that may arise while reading input.
Step 2 - Build the Huffman Tree
The Huffman tree is then constructed using the frequency table as a starting point. The C++ priority queue data structure can be used for this. The node with the lowest frequency will be at the top of the priority queue as the nodes are sorted by frequency. Then, by repeatedly extracting the two nodes with the lowest frequency, making a new node with their combined frequency, and re-adding it to the priority queue, you can make a binary tree. The root of the Huffman tree will be the last node in the queue.
Step 3 - Decode the Data
You can begin decoding the input data once the Huffman tree has been constructed. To accomplish this, you can traverse the Huffman tree from the root to a leaf node while reading the input data bit by bit. After decoding a symbol, you can output it to the output stream once you reach a leaf node. The traversal is then reset to the root, and the following symbol is then decoded.
Step 4 - Create Threads
The input data must be split up into smaller chunks that each thread will decode in order to make the decoder multithreaded. The C++ std::thread class can be used to create threads.
Step 5 - Divide the Input Data
The input data will now be split into pieces that each thread will decode. This can be accomplished by determining the size of each chunk based on the quantity of threads and the overall size of the input data.
Step 6 - Decode Data in Parallel
After splitting up the input data and establishing the threads, you can begin decoding the data simultaneously. Each thread will decode the chunk of input data that it has been given by iterating through the Huffman tree and writing the decoded symbols to a common output stream. For the threads to be synchronised, race conditions and deadlocks must be avoided.
Step 7 - Combine the Results
You can combine the results after each thread has finished decoding its assigned chunks by reading the output stream and writing the decoded symbols to the output file.
Testing and Debugging
The multithreaded Huffman decoder should be thoroughly tested after implementation to make sure it functions as intended. This can be accomplished by developing a test suite that contains test cases for various input data sizes and thread counts.
To make sure that the decoder can gracefully handle errors and exceptions, you should also test it using corrupted data. A debugger can be used to trace the execution and identify the issue if you run into any bugs or errors while testing.
Multithreaded programme debugging can be difficult, but with the right tools and methods, you can find the problems and resolve them. To debug your code and find problems like data races, deadlocks, and memory leaks, you can use tools like gdb, Valgrind, and Helgrind.
Conclusion
In conclusion, having a solid understanding of both multithreading and the Huffman coding algorithm is essential for writing an assignment on a multithreaded Huffman decoder in C++. Reading the input, constructing the Huffman tree, decoding the data, creating threads, dividing the input data, and decoding the data in parallel are the main steps in designing and putting into practice a multithreaded decoder.
A high-performance, multithreaded decoder that can process a lot of data can be made with the right approach and attention to detail. Additionally, by following the instructions in this blog, you can produce a striking assignment that showcases your programming abilities as well as your familiarity with multithreading and data compression.
I hope this blog has been helpful in guiding you through the process of writing an assignment on a multithreaded Huffman decoder in C++. Good luck with your project!